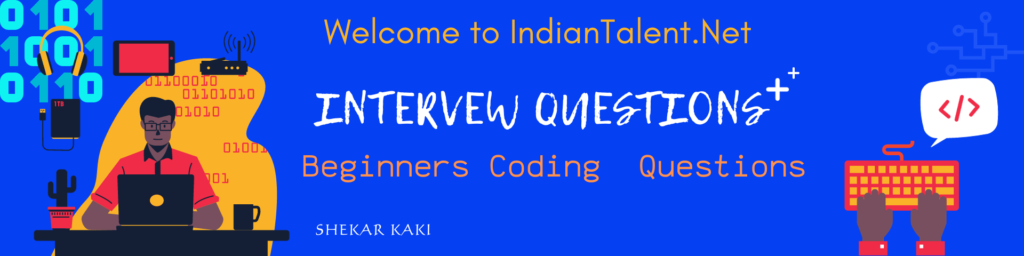
YouTube.com
“Python is fast enough for our site and allows us to produce maintainable features in record times, with a minimum of developers,” said Cuong Do, Software Architect, YouTube.com.
Entering the realm of coding interviews can be a daunting experience, especially when it comes to mastering Python—an immensely popular programming language. Whether you’re fresh to the world of coding or looking to land your first tech job, preparing for Python coding interviews is an essential step towards success.
Here, we’ll delve into a series of fundamental Python coding interview questions tailored for beginners. Embrace these inquiries as stepping stones to strengthen your Python skills and ace your upcoming interviews.
1. What is Python?
Answer: Python is a high-level, interpreted programming language known for its simplicity and readability. It emphasizes code readability and allows developers to express concepts in fewer lines of code compared to other languages.
2. Explain the Difference Between Lists and Tuples.
Answer: Lists and tuples are both sequence data types in Python. However, the primary difference lies in their mutability. Lists are mutable, meaning their elements can be changed after creation, whereas tuples are immutable—once created, elements cannot be altered.
3. How Does Python Handle Memory Management?
Answer: Python employs an automatic memory management system known as garbage collection. It manages the allocation and deallocation of memory, automatically freeing up memory occupied by objects that are no longer in use, thereby enhancing efficiency.
4. What Are Python’s Built-in Data Types?
Answer: Python offers several built-in data types, including integers, floats, strings, lists, tuples, dictionaries, and sets. Each serves a distinct purpose and facilitates various operations within Python.
5. Differentiate Between ‘==’ and ‘is’ Operators in Python.
Answer: The ‘==’ operator compares the values of two objects, determining if they are equal. Conversely, the ‘is’ operator checks whether two variables point to the same object in memory.
6. Explain the Concept of Python Decorators.
Answer: Decorators in Python are functions that modify the behavior of other functions. They enable a clean and efficient way to alter or extend the functionality of functions without changing their actual code.
7. What Is PEP 8?
Answer: PEP 8 stands for Python Enhancement Proposal 8. It’s a style guide that outlines the recommended coding conventions for writing Python code, emphasizing readability and consistency.
8. How Does Exception Handling Work in Python?
Answer: Exception handling in Python involves the use of try, except, and finally blocks. It allows the identification and management of errors or exceptional situations that may arise during code execution, thereby preventing abrupt program termination.
9. Explain the Purpose of ‘if name == “main“:’.
Answer: This line of code checks whether the script is being run directly or imported as a module. It helps in executing specific code only when the Python file is run directly.
10. Describe the Use of ‘lambda’ Functions in Python.
Answer: Lambda functions are anonymous functions defined using the ‘lambda’ keyword. They are concise and used for simple operations, often employed in situations where a function is needed for a short period without assigning it a name.
Remember, practice is key when preparing for coding interviews. Alongside theoretical knowledge, hands-on coding and problem-solving will significantly boost your confidence. Utilize online platforms, coding challenges, and projects to reinforce your understanding of Python concepts and ace those interviews with ease.
Python Coding Interview Questions for Beginners
Mastering Python interview questions for beginners sets a strong foundation for your coding journey. Embrace the learning process, stay persistent, and I am hear to help you to crack Python coding interviews effortlessly.
Beginner Level:
- Sum of Digits: Write a Python function to calculate the sum of digits in a given integer.
- Palindrome Check: Create a function to determine if a string is a palindrome.
- Factorial Calculation: Write a program to find the factorial of a number using both iterative and recursive approaches.
- FizzBuzz: Implement the FizzBuzz problem: print numbers from 1 to n, but for multiples of 3, print “Fizz,” for multiples of 5, print “Buzz,” and for multiples of both 3 and 5, print “FizzBuzz.”
- Reverse a String: Develop a function to reverse a given string without using any built-in Python reverse functions or slicing.
1.Sum of Digits: Write a Python function to calculate e sum of digits in a given integer.
# To find sum of digits inside given number
number = input("Enter a number to check sum of it : ")
# Convert the number to its absolute value to handle negative numbers, and cast to int
number = abs(int(number))
def sum_of_digits(number):
# Initialize sum to zero
digit_sum = 0
while number > 0:
digit = number % 10
# Add the extracted digit to the sum
digit_sum += digit
# Remove the last digit from the number
number //= 10
return digit_sum
print(sum_of_digits(number))
Explanation:
- This function calculates the sum of digits in a given integer.
- It starts by initializing
digit_sum
to zero. - It ensures that the number is positive by using
abs()
to handle negative integers. - It then iterates through each digit in the number using a
while
loop. - Inside the loop, it extracts the last digit using the modulo
%
operator, adds it to thedigit_sum
, and removes the last digit using floor division//
. - Finally, it returns the sum of the digits.
2.Palindrome Check: Create a function to determine if a string is a palindrome.?
def is_palindrome(s):
# Remove spaces and convert the string to lowercase
s = s.replace(" ", "").lower()
# Compare the string with its reverse
return s == s[::-1]
Explanation:
- The
is_palindrome
function checks whether a given string is a palindrome. - It starts by removing spaces and converting the string to lowercase using
replace()
andlower()
methods. - It compares the modified string
s
with its reverses[::-1]
using slicing. - If the original string is equal to its reverse, it returns
True
, indicating that the string is a palindrome; otherwise, it returnsFalse
.
3. Factorial Calculation (Iterative & Recursive):
# Iterative approach
def factorial_iterative(n):
factorial = 1
if n < 0:
return "Factorial does not exist for negative numbers."
elif n == 0:
return 1
else:
for i in range(1, n + 1):
factorial *= i
return factorial
# Recursive approach
def factorial_recursive(n):
if n < 0:
return "Factorial does not exist for negative numbers."
elif n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
Explanation:
- The
factorial_iterative
function calculates the factorial of a number using an iterative approach by multiplying numbers from 1 ton
. - The
factorial_recursive
function calculates the factorial of a number using a recursive approach by breaking down the problem into smaller subproblems.
4. FizzBuzz:
def fizzbuzz(n):
for i in range(1, n + 1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
Explanation:
- The
fizzbuzz
function prints numbers from 1 ton
. - For multiples of 3, it prints “Fizz”; for multiples of 5, it prints “Buzz”; for multiples of both 3 and 5, it prints “FizzBuzz”; otherwise, it prints the number itself.
5. Reverse a String:
def reverse_string(input_str):
reversed_str = ""
for char in input_str:
reversed_str = char + reversed_str
return reversed_str
Explanation:
- The
reverse_string
function reverses a given string without using built-in reverse functions or slicing. - It iterates through each character in the input string and constructs the reversed string by appending each character in reverse order to the
reversed_str
variable.
Above given Python code snippets cover various basic programming concepts such as factorial calculation, FizzBuzz implementation, and string reversal. They aim to provide clear guidance and understanding for beginners and serve as a valuable resource for learning Python programming basics.